Apart of all other useful things, Krita offers a support of execution of Python scripts. The possibilities include manipulating colors, changing document properties, manipulating layers, and more. The scripts can be executed via Scripter, saved to 10 Scripts, registered as an Action with Extension, or even assigned to a button via custom Docker.
Introduction
This is the first record in the series of articles around Krita Scripting. The entire series consists of the following material:
- How to start with Krita Scripting
- Krita Scripting main module
- Data Structures and Functions
- Classes and Objects
- Krita Scripting Components
- Operations with Layers
- Apply Filters
In this record, our focus will be learning the basics of Python itself, and the simplest way possible for executing the scripts. Other options how the scripts can be made permanent in your app we will discuss in the following records.
Scripter
Let’s start simple firsts. The Scripter. Open you Krita application and create a new Document. The parameters of the document are not important. Via menu choose Tools – Scripts – Scripter. Once you open the Scripter you should see the following window
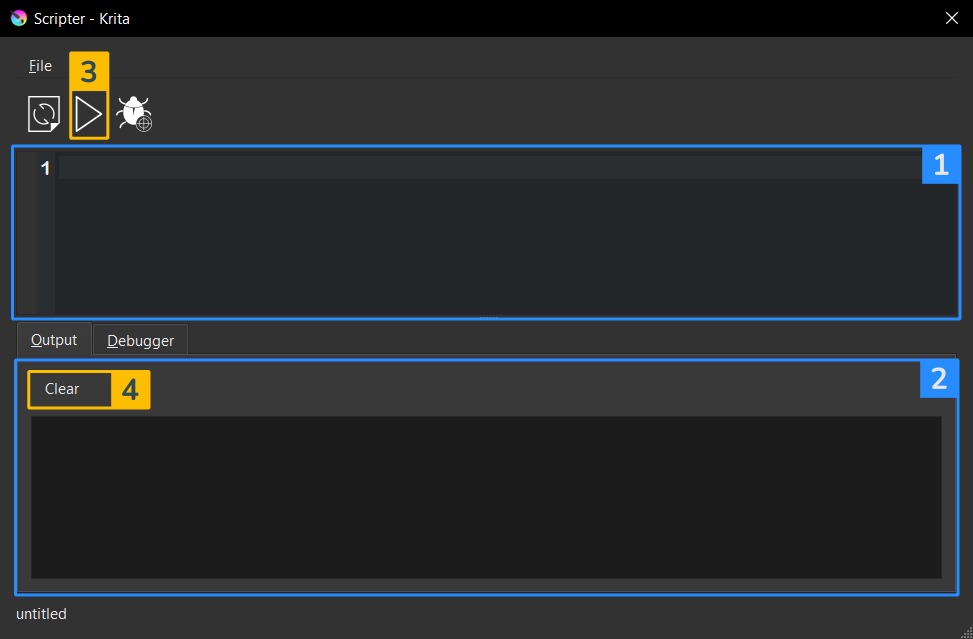
There are four aspects of the window that is interesting to us (labeled accordingly).
The first, the area where the script is being written. Here you will spend the most of the time. It is the place where you input your Python commands.
The second, the output area, it will contain all the messages that will be printed by the functions you call or by using the print function.
The third, Run button, by pressing this button you will trigger the script execution. We will talk about it in a bit.
The fourth, clear button. It will be helpful when you want make sure you see proper output and do not confuse with the previous runs.
Type the following command into the input area and press Run:
print("Hello World!")
Congratulations! You just created your first script. Welcome to the Python world. Let’s now learn the basics, how the Python language works.
Btw. You should see the message “Hello World!” in the output area. You can ignore the warning for now.
Python Basics
Let’s start with discussion what the Python program is. Python is considered to be an interpreting programming language. That means that all the commands in the program are being read and executed line by line.
Try running these two commands:
print("Hello World!") print("Hello Planet!")
Notice that the output shows the “Hello World!” first, then the “Hello Planet!”. Try to change the order of lines and see what happens.
This is important aspect to know once we talk about variables.
The Python program (or often referred as script) is the sequence of python commands. When program is executed the interpreter reads each line and updates its internal state. Then things defined in the first line might be used in the second line. That is why order of operations is important.
Building Blocks
There are multiple aspects of the Python that are important to learn. We start with variables and operations. We also touch functions. In the further records we will learn about other building blocks. They are control statements, classes, data structures and modules.
You can imagine a variable as a basket. We put the value we are interested in this basket. Then we assign a label to this basket (for instance by attaching a tag). We can then find that basket by the tag and access its content – the value. When we mention the variable in our script for the first time we call it variable definition. When we “put value in the basket”, we call it value assignment.
Here the example. We create a variable (called color) and assign a value “green”.
color = "green" print(color)
Try to copy and paste this code into your Scripter window and run it. See what will happen. It is important to replace the code.
We encourage you to copy and paste all the code pieces you encounter to learn how they work.
We can define color by its three components: r, g, and b:
r = 0 g = 255 b = 0 print(r, g, b)
Try to change the order of the print and variable definition and see what happens.
You noticed when we used words we put the word into quotes. This is the way to tell the Python that the value we will have in the variable will be text. However, if the value is the number, we just set the value as number.
This difference in what kind of things is stored in the basket is called a data type. The text in quotes is called a string, the values such as 1, 2 and 3 are integers. If the value has a floating point (like 1.618) then this is the float. The data types can also include more complicated things like lists, dictionaries or even custom types. We will learn how it works in the following records.
Once the variable is defined, we can perform operations with it. Different types of variables support different kind of operations. All the numerical types support all arithmetic operations like plus, minus, multiply, etc.
Let’s say we have a variable that controls our color HUE (let it be hue). We can shift the value of the variable by adding or substracting the value.
hue = 132.34 print(hue) hue -= 60 # this is identical to `hue = hue - 60` print(hue)
Here, we start with hue value of green color and shift it towards the yellow.
Notice we used the strange “hashtag” symbol (hash). This is special symbol in Python that tells the interpreter to ignore anything that goes after this hash symbol. There is also a weird looking quotes. You may ignore it for now. This is something that developers use to highlight piece of code in the Markdown documents.
The important thing is we perform an operation of subtraction of number 60 from the variable hue and set this value back to hue.
Functions
One last component of the Python programming that we will discuss in this record is function. Functions can be considered as a small program that is designed to do one single task. We already encountered one of the functions. That is print. The task of the print function is to accept input and (as the name suggests) print it to the output. Python supports large variety of functions, they include summing numbers, checking the truthiness of the list (the data structure we will discuss in further records), finding the maximum between two numbers, doing operations with files and more.
Broadly speaking the function is an operation that can take an input and produces an output. We call input of the functions an argument. And what the function returns is a result.
Here is an example of function that finds the maximum between two numbers:
a = 5 b = 3 result = max(a, b) print(result)
You should get the number 5 as your output.
This function has two arguments a and b. The result of the function will be the number that is larger than another one.
Further Reading
Python can be intimidating at first. However, if you take one step at a time, your will be able to learn all the aspect you need to improve you work with small scripts.
Despite of the content of this tutorial series you can always refer to these resources to learn python:
- Python Documentation – reliable place to learn how some of the aspects of the programming language is operating.
- Udemy Course – If you like to learn from the videos, this course is thus far one of the most useful to start with Python.
- Python Crush Course Book – If you are the book person, we would recommend to use this one to learn all the aspects you need.
Word about Chat GPT
It might be tempting to ask ChatGPT to generate scripts for you. However, you are signing yourself up to something that you do not understand and potentially inviting some security threats for your computer.
Keep in mind that all the script you run in the scripter can do pretty much anything on your PC. That is why it is important to learn the language first. At lest to the point when you can tell that you understand everything that is going on in the script generated by the generative model.
Next
Now you are ready to learn about Krita module and start using basic Krita operations with scripts.
Feedback and Suggestions
Should you have any questions or maybe you want to suggest next topic to cover. Please join our community and let us know.