The plugin comes with a set of functions and classes. This API enhances user capability in quick scripting.
Keystrokes
Sometimes it is required to trigger a Keyboard shortcut that might impact the tool outside the Krita application. One of the example is the usage of FanzyZone. By setting a keystroke user can automate the placement of the application. Learn more here. This functionality is available only on Windows.
send_keystroke
Generate keystroke like “Ctrl+J” by providing the string with a keystroke. The keystroke is created by writing a sequence of keys in plus notation. Example:
from actuator.api.keystroke import send_keystroke
send_keystroke("Ctrl+J")
List of supported keys:
‘LMButton’, ‘RMButton’, ‘MMButton’, ‘Backspace’, ‘Tab’, ‘Enter’, ‘Shift’, ‘Ctrl’, ‘Alt’, ‘CapsLock’, ‘Esc’, ‘Space’, ‘PgUp’, ‘PgDn’, ‘End’, ‘Home’, ‘Left’, ‘Up’, ‘Right’, ‘Down’, ‘Ins’, ‘Del’, ‘0’, ‘1’, ‘2’, ‘3’, ‘4’, ‘5’, ‘6’, ‘7’, ‘8’, ‘9’, ‘A’, ‘B’, ‘C’, ‘D’, ‘E’, ‘F’, ‘G’, ‘H’, ‘I’, ‘J’, ‘K’, ‘L’, ‘M’, ‘N’, ‘O’, ‘P’, ‘Q’, ‘R’, ‘S’, ‘T’, ‘U’, ‘V’, ‘W’, ‘X’, ‘Y’, ‘Z’, ‘LWin’, ‘RWin’, ‘-‘, ‘.’, ‘/’, ‘F1’, ‘F2’, ‘F3’, ‘F4’, ‘F5’, ‘F6’, ‘F7’, ‘F8’, ‘F9’, ‘F10’, ‘F11’, ‘F12’, ‘F13’, ‘F14’, ‘F15’, ‘F16’, ‘F17’, ‘F18’, ‘F19’, ‘F20’, ‘F21’, ‘F22’, ‘F23’, ‘F24’
Keep in mind that the keys has to be listed in a shortcut exactly as in the list above.
User Interface
Is a bulk part of the API. With these symbols user will be able to quickly construct interactive sequence without much of a coding.
Dialogs
ConfirmItemsListDialog
Provide the list of items and the message with the action and prompt the user to confirm with Yes or reject with No.
from actuator.api.ui.dialogs.confirmitemslist import ConfirmItemsListDialog
animals = ["dog", "cat", "raccoon"]
dialog = ConfirmItemsListDialog(items=animals, msg="Are these all animals?", title="Zoo")
dialog.exec()
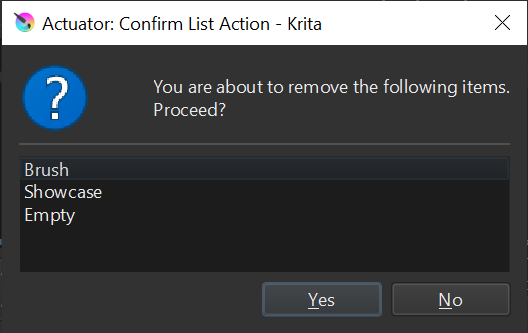
CustomIntDialog
Create this dialog to extract the integer value from a user.
The constructor of a class has three input arguments:
- min_value: Minimum value for the number in range.
- max_value: Maximum value for the number in range.
- mgs [default=””]: The message to display next to input value.
Once the dialog is executed a field value will contain selected value. If the user will click “Cancel” or closes the window the value will be equal to None.
from actuator.api.ui.dialogs.customint import CustomIntDialog
dlg = CustomIntDialog(-15, 15, "Choose the angle")
angle = 0
if dlg.exec():
angle = dlg.value
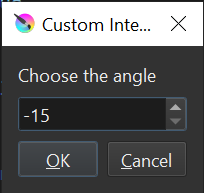
SelectItemsDialog
Let the user select one or multiple items from provided list.
The constructor of a class has three input arguments:
- items: List of string to select from.
- msg [default=””]: The message to display on top of the dialog.
Once the dialog is executed a field value will contain the list of indices for selected items. If the user will click “Cancel” or closes the window the value will be equal to None.
from actuator.api.ui.dialogs.selectitems import SelectItemsDialog
brushes = ["b) Basic-5 Size", "c) Pencil-1 Hard", "d) Ink-2 Fineliner", "f) Charcoal Rock Soft"]
dlg = SelectItemsDialog(brushes, "Select your favorite brushes")
if dlg.exec():
favorite = dlg.value
The interface of the window will also contain two buttons: Toggle All On and Toggle All Off to trigger all the checkboxes.
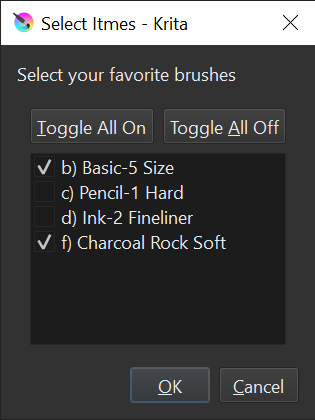
get_selected_items
Provide the function with a list of items and get list of selected indices
The function has one input argument:
- items – the list of strings to use a items to select.
If the dialog is closed the function will return empty list.
from actuator.api.ui.dialogs.quick import get_selected_items
brushes = ["b) Basic-5 Size", "c) Pencil-1 Hard", "d) Ink-2 Fineliner", "f) Charcoal Rock Soft"]
favorite = get_selected_items(brushes)
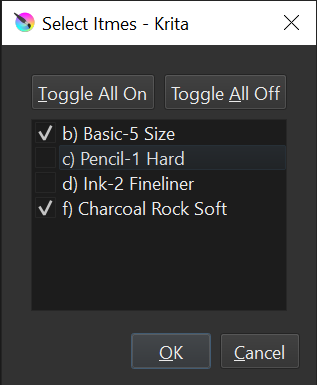
get_color_with_dialog
Show color-picker dialog to select color
from actuator.api.ui.dialogs.quick import get_color_with_dialog
color = get_color_with_dialog()
Keep in mind that the color selection dialog is OS specific.
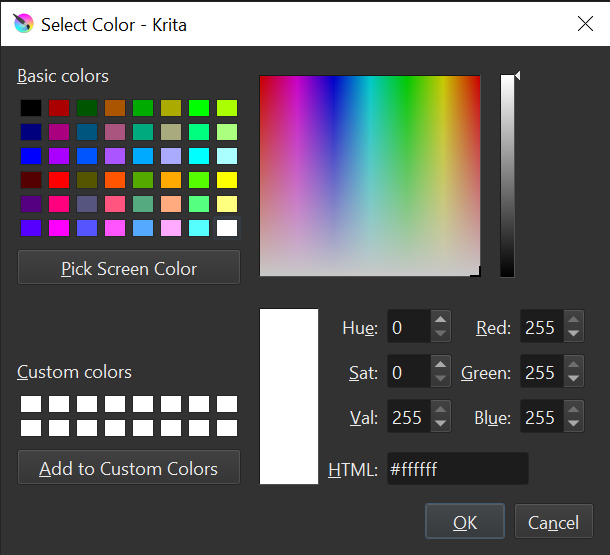
Filters
Even though the action with filter can be executed as a script step, you might need to ask the user to set the filter settings. Actuator API has a large number of filter dialogs implemented and ready to be used.
from actuator.api.ui.dialogs.filters import *
- ColorToAlphaDialog
- GaussianBlurDialog
- UnsharpDialog
- BlurDialog
- BurnDialog
- ColorBalanceDialog
- ColorTransferDialog
- DesaturateDialog
- EdgeDetectionDialog
- EmbossDialog
- GaussianHighPassDialog
- GaussianNoiseReducerDialog
- HeightToNormalDialog
- LensBlurDialog
- MotionBlurDialog
- RandomNoiseDialog
- OilPaintDialog
- PixelizeDialog
- PosterizeDialog
- RaindropsDialog
- RandomPickDialog
- RoundCornersDialog
- SmallTilesDialog
- ThresholdDialog
- WaveDialog
- WaveletNoiseReducerDialog
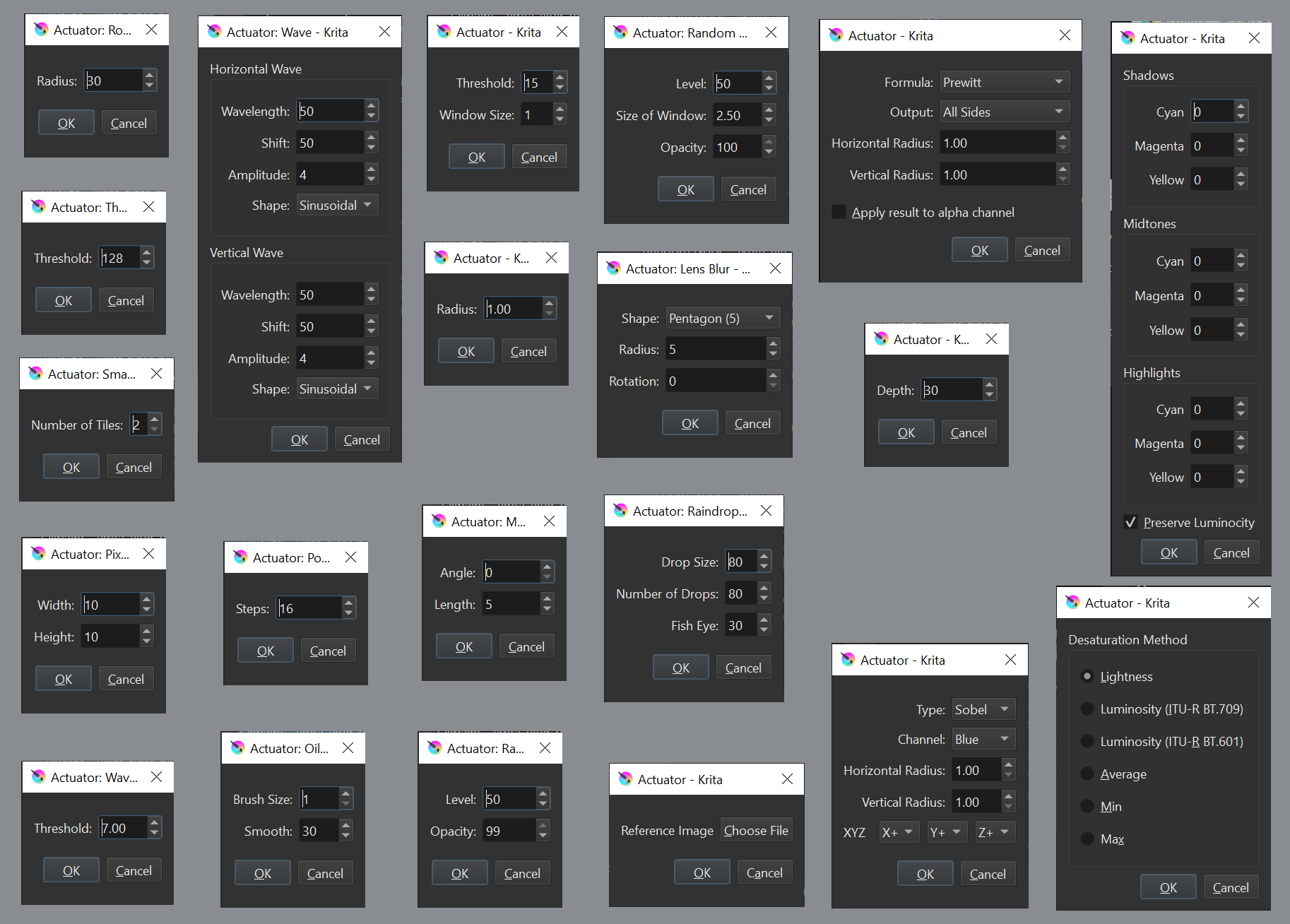
Widgets
SearchableListViewSearchableListView
Convenient UI component to display a list of item with or without icon. Also contains an input box to filter items in a list.
from actuator.api.ui.widgets.searchablelist import SearchableListView
items = [
"Tango", "Alpha", "Bravo", "Charlie", "Some", "More", "words", "to", "match",
"The",
"Search",
]
lw = SearchableListView(items, read_only=True)
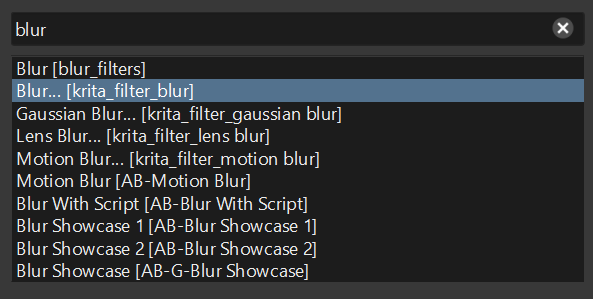
SquareButton
Convenient QPushButton that stays square. The base size is the height of the button. Useful to make panel buttons.
from actuator.api.ui.widgets.squarebutton import SquareButton

Actions
Scripting alternatives to built-in actions.
Action
Replica of Krita.instance().action(“”). The differences is that these actions are blocking. So when you call them the script will finish the action first. Then proceed to next operation.
from actuator.api.actions import Action
dup = Action("duplicate")
dup.trigger()
Following actions are implemented:
- deselect
- duplicate
Color
Functionality to manipulate colors
from actuator.api.color import *
Functions:
- rgba_to_hexa – Convert RGBA (123, 245, 123, 210) to HEX with alpha (#945d5dff)
- rgba_to_hex – Convert RGBA (123, 245, 123, 210) to HEX without (#945d5d)
- hex_to_rgb – Convert HEX color #945d5d to RGB value (231, 12, 54)
- rgb_to_hsl – Convert RGB to HSL
- hex_to_hsl – Convert HEX color string to HSL
- rgb_to_hsv – Convert RGB to HSV
- hex_to_hsv – Convert HEX string to HSV
Utils
halt
Wait for some time before continuing operations.
from actuator.api.utils import halt
wait_three_seconds = 3000
print("Before")
halt(wait_three_seconds)
print("After")
state
from actuator.api.utils import state
state["counter"] = state.get("counter", 0) + 1
print(state["counter"])
Pure python dictionary. Useful to save state between sequence executions. Preserves the state only during application runtime. After closing the application the state is lost.
Neat thing about state is it allows to create an action step that cycle though set of tools or brushes. Here the example.
diplay_global_message
Show a little popup on the left bottom of a screen. Helpful to inform the user from within the code. Every time the function is called the messages are put into the queue and will be displayed one after another. If the user clicks anywhere on a screen, the message will disappear and the next message (if any) will be displayed.
from actuator.api.global_message import diplay_global_message
def run():
diplay_global_message("info", "My cookies is ready", 20)
diplay_global_message("warning", "Your cookies are cooking", 3)
diplay_global_message("error", "And you... You are not getting any cookies!", 4)
diplay_global_message(
"info",
"To make the dialog appear with a fade-in animation of 500ms, you can use Qt's animations and set the window opacity to gradually increase over time. Here's the modified code to achieve this effect",
4,
)
run()
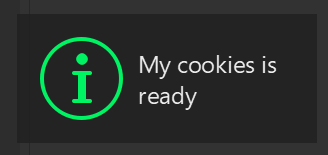