To achieve this effect with Actuator we will need a two step sequence. First step will merge the layer and store the blending mode. The second step will apply the blending mode to the merged layer.
Step 1:
from krita import Krita, Node
from actuator.api.utils import state
from actuator.apicandidate.utils import hopeful_wait
doc = Krita.instance().activeDocument()
node: Node = doc.activeNode()
bm = node.blendingMode()
state["blending-mode"] = bm
node.mergeDown()
Step 2:
from krita import Krita, Node
from actuator.api.utils import state
from actuator.apicandidate.utils import hopeful_wait
doc = Krita.instance().activeDocument()
node: Node = doc.activeNode()
bm = state["blending-mode"]
node.setBlendingMode(bm)
We need to separate the steps to give the Krita some time to complete the merging operation. The Actuator will handle the waiting time for us.
To achieve the same result with 10 scripts a bit of modified version of the script will be needed. Here it is:
from krita import Krita, Node
from PyQt5.QtCore import QTimer
timer = QTimer()
timer.setInterval(150)
timer.setSingleShot(True)
def set_blend(mode):
doc = Krita.instance().activeDocument()
node: Node = doc.activeNode()
node.setBlendingMode(mode)
def run():
doc = Krita.instance().activeDocument()
node: Node = doc.activeNode()
bm = node.blendingMode()
node.mergeDown()
timer.timeout.connect(lambda x=bm: set_blend(x))
timer.start()
run()
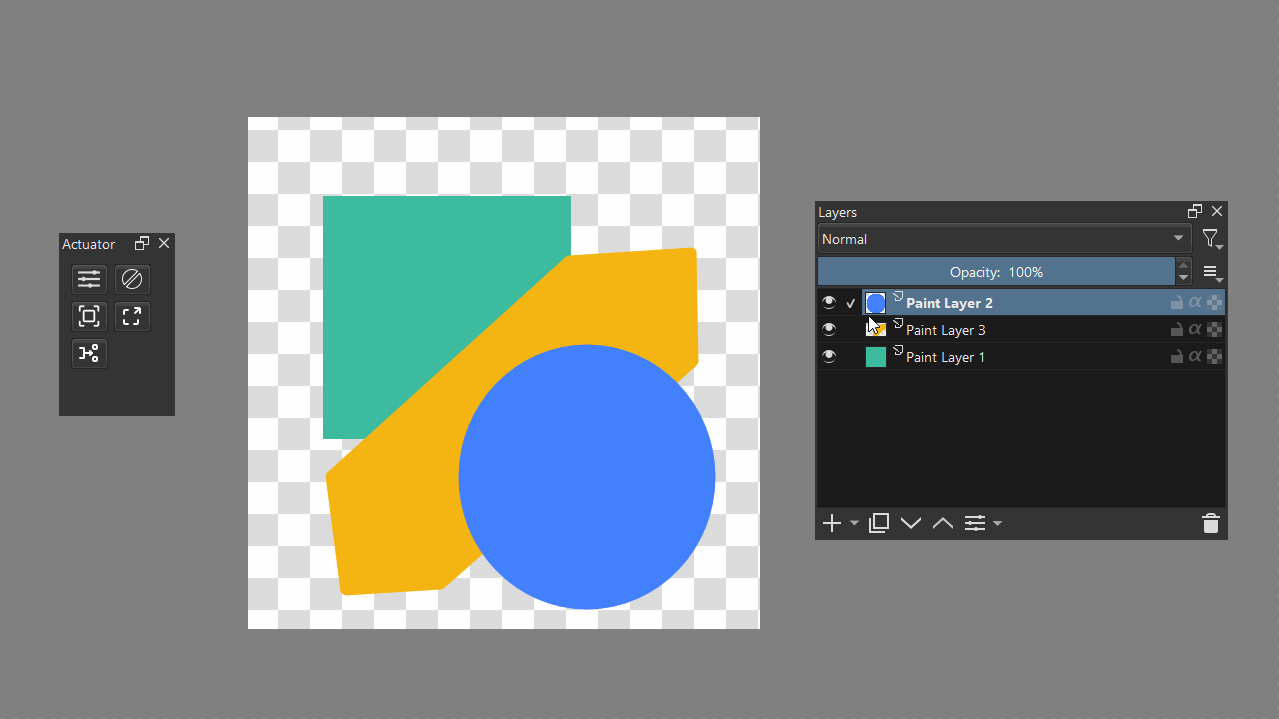
Download this sequence.